We know that our Shopify App Developer community has several JavaScript fans, and we’re big fans of some of its frameworks too, like React.
As the JavaScript community continues to build and innovate, it feels like anything dot JS exists. Among the many new frameworks however, Vue.JS has been among the most buzzworthy in the community. In fact, not only is it a wildly popular open source project, it’s also the second most popular JavaScript framework on GitHub.
To help you level-up your skill set and understand what this framework is all about, we recently partnered with Cassidy Williams, Senior Software Engineer at L4 Digital, to show you the ins and outs of Vue.js and how it can be used to rapidly build front end experiences for web apps.
Before we dive into a re-cap of Cassidy’s steps for getting started with Vue.js, here’s a little background on why it was created, and how it differs from other popular frameworks, like Angular and React.
Origin of the Vue.js framework
Launched publicly on GitHub in February 2014, Vue.js was brought to life by a former Google developer, Evan You. He had long been attracted to JavaScript since it enabled a straightforward way to build something and share it instantly. After being recruited by Google, a lot of his time was spent on in-browser prototyping. His team worked to build things that were quick to set-up, but still tangible. For this purpose, a framework they sometimes used was Angular.
While Angular helped speed things up, offering data binding to deal with the DOM without having to touch it directly, there were still extra concepts that demanded code be structured a certain way. In an effort to further simplify the coding process and help developers move faster, Evan worked to build something that had these efficiencies, but was even more lightweight.
You might also like: The Essential List of Resources for Shopify App Development.
Getting started with Vue.js
Vue.js was designed to be approachable, so that anyone with a basic knowledge of HTML, JavaScript, and CSS could easily get started with this framework. There are also many great beginner templates you can get started with.
After choosing which API you want to build on top of, visit the Vue.js repository, open your consoles, and get coding! You’ll begin by installing the NPM globally, then loading the CLI:
npm i -g vue-cli
Then, initialize Vue to start a new project, and choose a starter project. In this demo webpack
was used.
vue init webpack
Once the project is initialized, you’ll fill-in all the details, such as name, project description, and author. You can also choose the Vue build. In Cassidy’s tutorial, the default build was used.
You’ll also install the router and specifying if you want to run tests — we opted for no
.
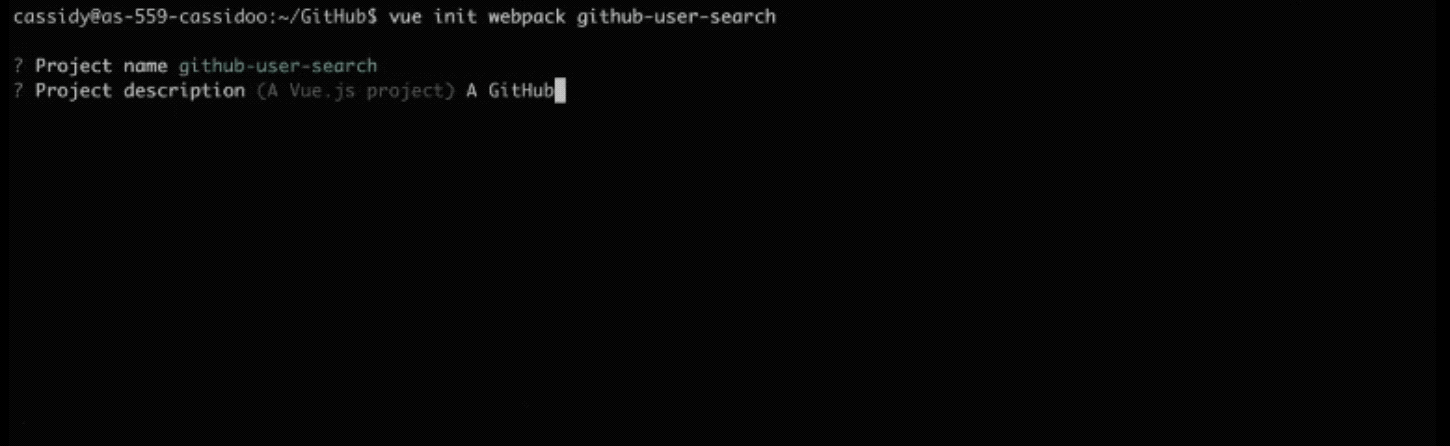
After these basics have been specified, you’ll use npm install
to install all the libraries Vue specifies you’ll need. Once that’s complete, you’ll use npm run dev
to start the dev server. Voila — you now have a basic web app UI!

Now that we have a live app, it’s time to get under the hood and customize it.
You might also like: [Free Webinar] How to Build Your First Shopify App.
Customizing the app UI
As with most web apps, there’s an index.html. Among all this code, the entire app lives within the one little line <div id="app">/div>
. In fact, if you wanted to make a standalone vue app that lived within a larger web app, you could do so within this app div.

If you also want to take a peek at all the libraries Vue gives you, you can do that in package.json
.

Now onto main.js
aka the main JavaScript. Here it imports Vue, the app, and the router. Also specified is that the router uses the app templates and pulls in the app components. This is what makes up the backbone of your app.

Now in the app component — app.vue
— you can view the structure and style. Note that app.vue
is a Vue.js file, and a Vue file always contains:
- A template
- A script
- A style
In Vue, the given style defaults to being applied globally, but if you want it to become specific to the component itself, you’ll add scoped
with <style>
.
In the HelloWorld.vue
component, you can make changes here that will appear in the app’s user interface. To change the h1 component, for example, you would update the msg
.
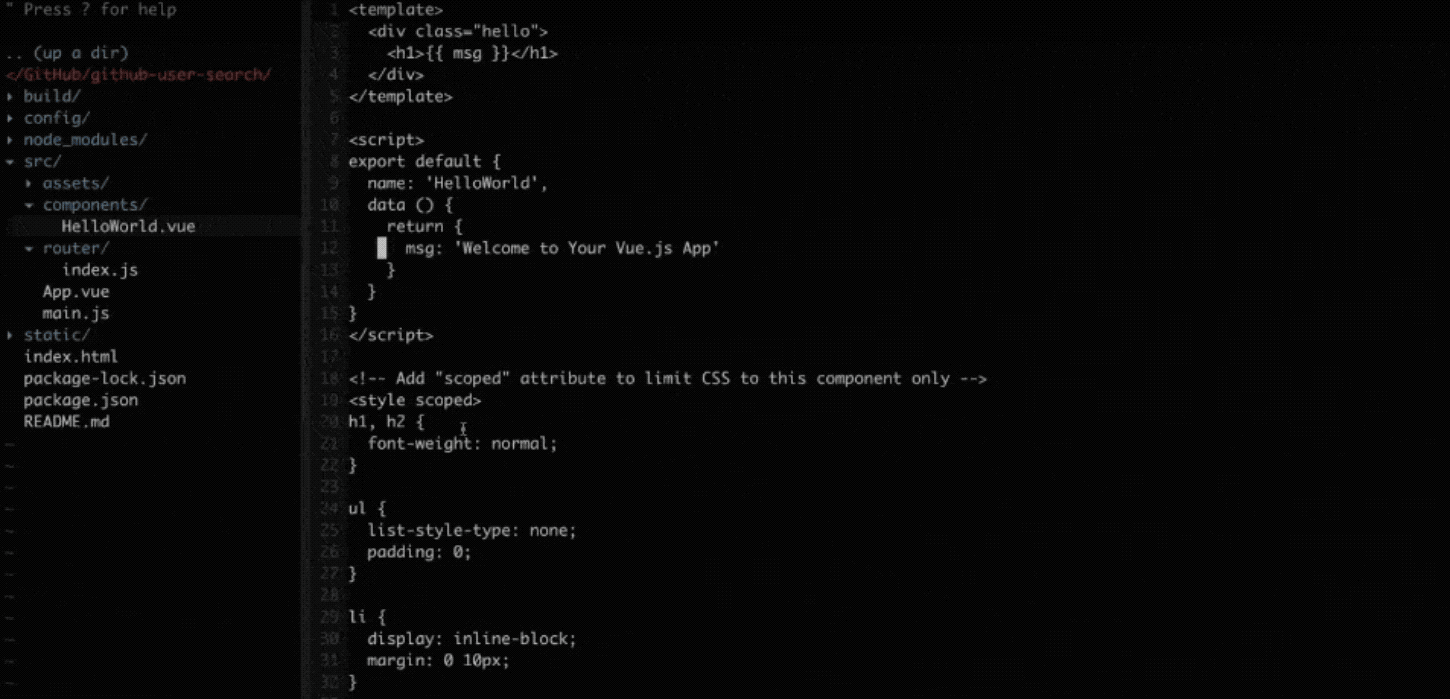
Now that you have the basics set-up, you can make the app more robust by adding a new route.
Using the router to make a new route
In the router, you’ll add a new component in index.js
. Following Cassidy’s tutorial, you can make your app bilingual and create a spanish version of the Hello World component, which we’ve suitably named the Hola Mundo component. The set-up for this would be similar to the HelloWorld coding that came within the webpack, except that you would specify a unique path, name, and component name:
After establishing this new component, you can copy and paste the existing code from HelloWorld
and update:
<div class='hola'>
name: HolaMundo
msg: 'Bienveindos a Vue!'
Then, make sure to import this new component into the router:
import Holamundo from '@/component/HolaMundo'
After this is initialized, a new page in your web app will be created.

Now that you have the two pages, you probably want them to link to each other. Unlike with a React router, where you have to add a link component, in vue you can use a simple a
component.
In the HolaMundo component you’ll add:
And in the HelloWorld component you’ll add:
When you refresh the app, both pages will link to each other! Since we now have the basics setup, let’s move onto building a more complex component.
Adding a functional component for counting
To show you how simple it can be to set-up an interactive component, Cassidy walked us through how to build an interactive counter with a button that added a count upon being clicked. Some of the same steps from above apply, but this time you’ll be making a button appear on the UI, programming a response to an action, and making sure that action is properly displayed within the app.
First, you can make the button. In the HelloWorld component, add:
<button></button>
Now add a new data attribute, count: 0
to make the counter start at zero. Now go back to your button and add the desired response to being clicked, as well as a directive:
<button v-on:click=”count++”>Increase the count!</button>
To display the counter in the app’s UI, you’ll add:
<div>[{count}]</div>
Just like that, your app has a counter!
Add user search function
The sample app Cassidy created for this tutorial is based around searching for different GitHub users. Now that you know the basics of setting up a view app and adding components, you have a good base for building this user search functionality.
First, create a new component:
:e “src/components/GitHub.vue”
After you save it, it will appear under components, along with HelloWorld and HolaMundo. Like before, copy and paste the code from an existing component, and update the component name and message.
Now that the component is established, the first thing to do is create a form with an input element:
Then, you’ll import this to the router to make it visible on the app UI. Now that it’s visible, it needs to be made functional. Since you want to create a search form, you’ll need to make a query function.
In Vue, when you want to have certain methods attached to a function you simply add methods:
.
In this instance, the method will be:
Now that data can be retrieved, it’ll need to be used and parsed. In the data, have a results object and have it start at null:
results: null
To print the results on the UI, and organize by name, user bio, and location, you’ll write the following lines:
Let’s get even fancier. To show the image associated with the search results:
<img v-bind: src=”results.avatar_url”/>
Last but not least, you can remove the Vue logo that was given to you with the webpack template, by finding it in the App.vue file, and deleting it. It’s also within this file that you can add image margins and sizing to format the image that displays with your GitHub search results.
And there you go — a web app for searching GitHub, made in under thirty minutes!

From opening your console to Hello World, in a flash!
When it comes to Vue.JS, simplicity and speed are the name of the game. As our recent guest webinar host Cassidy Williams demonstrated, even if it’s not your go-to framework, Vue.JS is great for the prototyping process, and when you need to quickly create a live and functioning web app front end on top of your backend.
Found this webinar helpful? Sign up to be notified when new webinars are announced, and continue to one-up your design and app building skills.