When selling online, one of the most requested product features is image zooming. Zooming is often used on a product image to enlarge it for better viewing by potential customers. There are a lot of different zoom libraries available online for free, and at the end of this article I’ll go into a few more options that you could use with Shopify themes.
In this tutorial, I’ve included two methods you can use to add zooming to your client’s site:
Be sure to use only one of these methods, not both. These are two separate tutorials, so choose whichever is best for you. Let’s dive in.
Grow your business with the Shopify Partner Program
Whether you offer marketing, customization, or web design and development services, the Shopify Partner Program will set you up for success. Join for free and access revenue share opportunities, tools to grow your business, and a passionate commerce community.
Sign up1. Zooming via jQuery
I’ve opted to go with an oldie, but a goodie, jQuery Zoom by Jack Moore. We currently use this plugin for zooming product images in the free Shopify theme, Debut. This zoom plugin has little to no styling (so you can customize this yourself), and is super simple to implement. What I like most about this plugin is that it has a clear changelog and is used by a lot of people online.
View Demo StoreDownload jQuery Zoom
This plugin is compatible with jQuery 1.7+ in Chrome, Firefox, Safari, Opera, and Internet Explorer 7+.
If you currently have a node set up for theming, you can install this plugin via npm by running:
npm install jquery-zoom
Or, you can download the jQuery Zoom plugin.
For more tools, check out our list of favorite Sublime text plugins.
1) Add jQuery if it doesn’t already exist
Most themes already have a version of jQuery running, and for this plugin to work you need a minimum version of 1.7+. What that means is that with most Shopify themes you probably won’t need to add jQuery, unless you’re building the theme from scratch. If you’re building an app and use jQuery, make sure to read through the documentation on how to use jQuery responsibly, in order to avoid conflicts with themes that already include it.
You can inspect your theme to see if jQuery is already being loaded, by either searching for the script or going to the Console tab in your inspector and running jQuery.fn.jquery
, which will return the version of jQuery the website is running.
Alternatively, you can also check the theme.liquid
to see if it’s being loaded, or already loaded in a vendor.js
file inside the scripts/
directory. Never load more than one version of jQueryon a website at a time, as it will increase the load time of pages and cause a slew of other JavaScript problems on your client’s site.
If you need to add jQuery to your theme, simply add the following line to the head of your html:
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.2.4/jquery.min.js" defer=”defer”></script>
2) Add the zoom.min.js plugin file to your theme
From the plugin folder you downloaded, add the minified jQuery Zoom plugin (jquery.zoom.min.js
) to your Assets folder. Then, in your theme.liquid
file, after where jQuery is being loaded, link the plugin file to your theme using the following Liquid code. Consider adding a check to include it only on product pages instead of on all pages (especially if it’s only being used on product pages):
{% if template contains 'product' %}
<script type="text/javascript" src="{{ 'jquery.zoom.min.js' | asset_url }}" defer=”defer”>
{% endif %}
Alternatively, you can also add the minified code to the end of a vendor.js
file—if you already have a theme that uses one—to avoid the extra request.
3) Edit product.liquid
to target the zoomed image
For the zoom plugin to work, it appends HTML inside the element it’s assigned to. Therefore, the element that it’s assigned to must accept html; the <img>
tag cannot contain other HTML elements, so it needs a container or wrapper element.
One of the easiest ways to do this is to add a wrapper around your main product image with JavaScript. In your product.liquid
template, or if you’re using sections, add the classes image-zoom
and data-zoom
to the main image for your product section. Note that the featured_image
for your product might be called something else, depending on the theme you’re using.
You’ll also want to use Liquid to generate the URL of your large-image (that will be your zoomed image) as a data-attribute. In this case I’ve named it data-zoom
. We’ll be able to use this later on in initialization, enabling you to pass the URL to the .zoom()
method as a URL property, specifying a larger version of the image (instead of loading one large one and scaling it down).
You might also like: What is Progressive Enhancement and Why Should You Care?
4) Initialize the zoom plugin dynamically
Because there are many different themes with a wide variety of CSS that could be applied to elements, the code below is triggered by the added image-zoom
class on the main product image. It will add a <span>
wrapper with jQuery that the zoom plugin will then append additional HTML to, creating the zoom effect. This will avoid any issues caused by CSS layouts, or other styles that might affect a manually created container.

There are many alternative ways of implementing this plugin, and depending on what other JS events you have triggering for product images, it might make sense to create a custom function like productImageZoom()
, and to also use the .trigger('zoom.destroy');
before triggering .zoom();
.
This would be the best approach if you’re using JavaScript to manipulate variant images, and loading a different feature-image based on which variant is clicked. For an example of this, take a look at how this zoom plugin is implemented in the Debut theme.
5) Customize your settings, and add styles as needed
The jQuery Zoom plugin comes with a bunch of other properties you can pass to the .zoom()
method. These can be found in the documentation for the plugin, and include on
, duration
, target
, touch
, and more.
You can also add styling to your zoomed images to give an indication that the image will zoom on mouseover
. If you’re using one of the other on
values like grab
, click
, or toggle
you might want to change the default cursor for image-zooming with some CSS.
For example, if you wanted to include something similar to the styling of the plugin demo, you could add the following styles, and the icon.png
into your assets folder:
You could also add styling that would change the cursor to indicate a grab-zoom option:
Note: Some of these CSS values only work in webkit enabled browsers; you can also provide an image for a custom cursor if you want them to be consistent in non-webkit enabled browsers.
For bonus points, consider extending the functionality we’ve already created using theme options, giving your client the option to turn zooming on and off at their leisure.
You might also like: 15 Tools to Customize Your Terminal and Command Line.
2. Zooming via vanilla JavaScript
For the vanilla Javascript option, we can use imgix’s Drift library which is lightweight and has no dependencies.
This library supports the latest version of Chrome, current and previous releases of Firefox, Internet Explorer, and Safari on a rolling basis, and the most recent minor version of the current and previous major release for the default browser on iOS and Android. I’ve picked this library because it’s well maintained and easy to implement.
If you currently have a node set up for theming, you can install this plugin via npm, by running:
npm install drift-zoom
Or, you can download the Drift library manually as well.
1) Add the Drift to your theme
Depending on your setup you can manually include the Drift dist in your theme. Note that this will appear on all pages if added through your theme.liquid
file, which can be less performant because it’s being included on all pages of your site. Consider adding a check to include it only on product pages instead of on all pages (especially if it’s only being used on product pages).
{% if template contains 'product' %}
<script type="text/javascript" src="{{ 'Drift.min.js' | asset_url }}" defer="defer"></script>
{% endif %}
Alternatively, you can also add the minified code to the end of a vendor.js
file, just like the jQuery instructions above—if you already have a theme that uses one—to avoid the extra request.
If you are using ES6, you can do the following to include drift in your project:
2) Edit product.liquid
to target the zoomed image
For drift zooming to work, it appends HTML inside an element with CSS positioning. If no element is specified to contain the zoomed image, then it will default to overlay on top and align with the closest positioned parent.
First, let’s add a wrapper around your main product image in your product.liquid
template, and inside that also add an empty div with a class of image-details
. If you’re using sections, you can instead add the data-zoom
attribute to the product section featured image. Note that the featured_image
for your product might be called something else, depending on the theme you’re using.
<div class=”image-container”>
<img class=”image-zoom” src=”{{ featured_image | img_url: '480x480' }}” alt="{{ image.alt | escape }} data-zoom="{{ featured_image | img_url: '1024x1024', scale: 2 }}>
<div class="image-details"></div>
</div>
You’ll also want to use Liquid to generate the URL of your large-image (that will be your zoomed image) as a data-attribute. In this case I’ve named it data-zoom
. We’ll be able to use this later on in initialization, to specify a larger version of the image.
3) Initialize Drift and set options
The next step is the basic setup, and is really straight forward:
Here we’re initializing Drift on the image by targeting the class of image-zoom
, then we’re setting the paneContainer
to the .image-details
div, in order to set the output of the zoomed content that will be rendered to that container.
You can also set up other more specific options for Drift, including offsets, zoom amount, enabling touch, and more. Those options can be found on the GitHub page for Drift.
4) Customize your settings and styles
The last step in this process would be to set up any additional styling you’d like to include for your image zooming with Drift. Instead of your zoom area covering the entire page, you can add styling so it’s set off to the side, or overlays other content on the page.
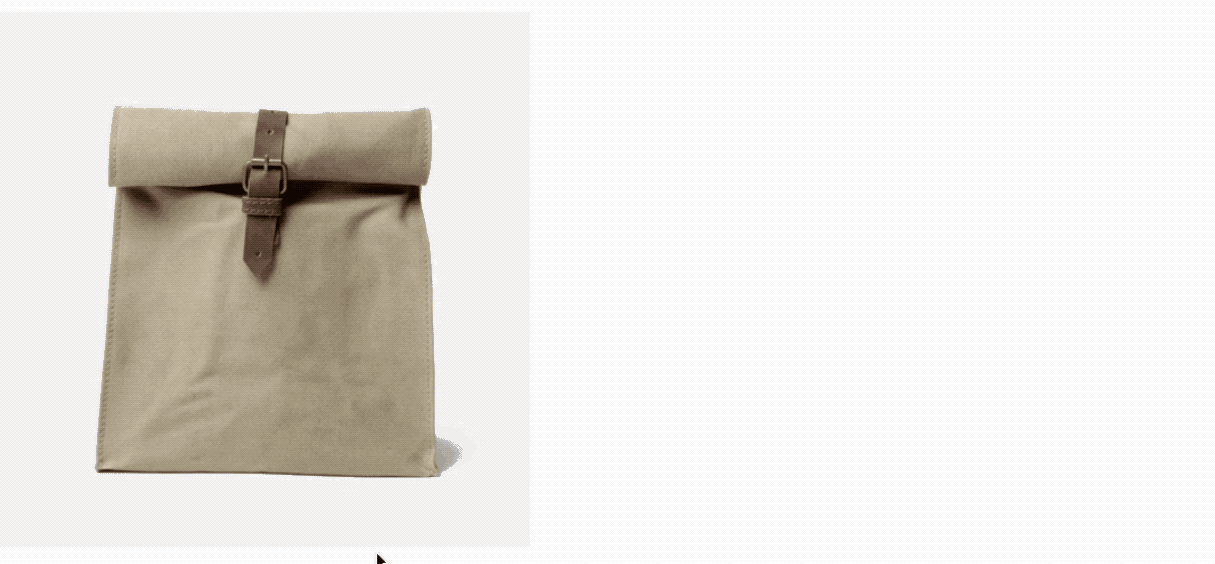
I’ve added some simple styles for two columns with CSS grid that you can see being used in the demo above:
Alternative plugins and Shopify Apps
If you’re not a fan of using the two options in the above tutorial, here are some alternatives to take a look at. I’ve also included a couple Shopify Apps specifically for product zooming.
EasyZoom plugin
EasyZoom is an elegant, highly optimized jQuery image zoom and panning plugin. It supports touch-enabled devices, and is easily customizable with CSS.
ZooMove plugin
ZooMove plugin developed with jQuery allows you to dynamically zoom images with mouseover, and view details with mouse move. Compatible with: jQuery 1.7+ in Chrome 42+, Firefox 41+, Safari 9+, Opera 29+, and Internet Explorer 9+.
In addition to these free plugins, there are also paid Shopify Apps you can install for your clients.
Magic Zoom Plus app
Magic Zoom Plus lets users hover to zoom an image and click to enlarge an image. It's one of the top-rated Shopify image zoom apps in the Shopify App Store, and works with mobile devices. There’s a free 30 day trial, and the app costs a one time price of $69.00.
Product Image Zoom By Gowebbaby app
Product Image Zoom By Gowebbaby is another image zoom app on the Shopify App Store, which uses a box or lens around your zooming area, making it feel like a magnifying glass inspecting a product. It sells for $3.99 per month, with a three day free trial.
You might also like: How to Create a Customizable Announcement Bar Section in Shopify.
Get zooming
Using a product zooming plugin can be a powerful tool for conversions, enabling customers to get up close and personal with the products your client is selling. By upselling a client and adding an additional functionality when building custom themes, you can add some extra billable hours to your project. Hopefully with the help of this article, adding that extra functionality will be a little bit faster. 🚀